An Introduction to Strings in Python
Understanding the Creation, Indexing, and Properties of Strings
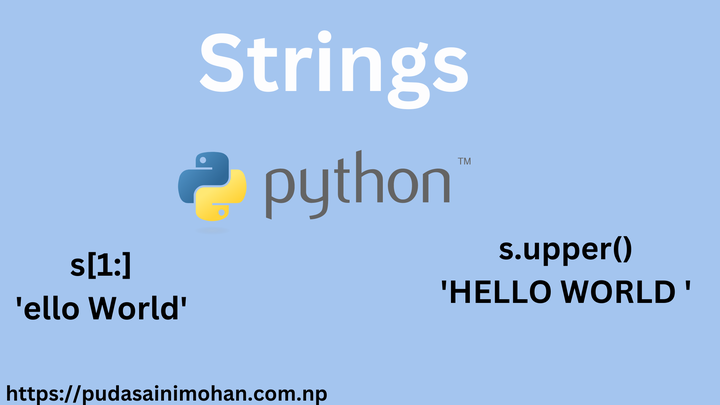
In Python, strings are a vital data type that play a significant role in storing text information, such as names, addresses, and other textual data. Strings are viewed as a series of characters, each with a unique position or index in the sequence. This allows us to easily access individual characters within a string using indexing.
For instance, the string “Nepal” is considered a sequence of five letters (‘N’, ’e’, ‘p’, ‘a’, ’l’) arranged in a specific order. With the help of indexing, we can extract individual letters from the string, such as the first letter ‘N’ or the last letter ’l’. This property of strings as sequences in Python makes them extremely versatile and useful for a variety of applications, including text processing and manipulation.
In this article, we will explore the creation, indexing, formatting, and various properties of strings in Python.
Creating a String
To create a string in Python you need to use either single quotes or double quotes. For example:
'Nepal'
'Nepal'
We can also use double quote
"Buddha was born in Nepal"
'Buddha was born in Nepal'
' I'm passionate about data science'
File "<ipython-input-4-da9a34b3dc31>", line 2
' I'm passionate about data science'
^
SyntaxError: invalid syntax
** error?**
The reason for the error above is because the single quote in I’m
stopped the string. You can use combinations of double and single quotes to get the complete statement or use backslash(\).
"I'm passionate about data science"
'I\'m passionate about data science'
'I'm passionate about data science'
Printing a String
The example above only displays one output despite having two lines of input. Is your assumption that it’s due to the identical sentences ? It’s important to note that simply entering a string in a Jupyter notebook cell will result in automatic output, but to display multiple strings properly, the print function should be utilized. to show both line we can do as follow
print("I'm passionate about data science")
print('I\'m passionate about data science')
I'm passionate about data science
I'm passionate about data science
We can use a print statement to print a string. here are some examples and their outputs:
print('Hello World 1')
print('Hello World 2')
print('Use \n to print a new line')
print('\n')
print('See what I mean?')
Hello World 1
Hello World 2
Use
to print a new line
See what I mean?
String length
In Python, you can find the length of a string using the len function. The len function returns the number of characters in a string. Here’s an example:
len('Hello World')
11
String Indexing
string indexing is a process of accessing individual characters in a string using an index. The index of a character in a string starts from 0, and the last character in a string has an index of len(string) - 1. You can access a character at a specific index in a string using square brackets []
. Let’s learn how this works.
Assign s as string and print it
s = 'Hello World'
Print(s)
'Hello World'
Show first element (in this case a letter)
s[0]
'H'
s[1]
'e'
We can use a :
to perform slicing which grabs everything up to a designated point. For example:
s[1:]
'ello World'
here, [1:] is a slice of the string that starts from the second character (index 1) and goes to the end of the string.
s[:3]
'Hel'
In this case, we tell Python to extract the characters from the first position (index 0) until the fourth position (index 3), but not including the character at the fourth position. This concept of “up to but not including” is a common occurrence in Python, and can be seen frequently in various statements and contexts. We can also use negative indexing to go backwards.
s[-1]
'd'
We can extract elements from a sequence by using index and slice notation, where the step size can be specified. To do so, we can use two colons followed by a number that represents the frequency of extraction. For example, to retrieve all elements with a step size of 2, we can use the following syntax.
s[::2]
'HloWrd'
Similary, We can use this to print a string backwards too
s[::-1]
'dlroW olleH'
String Properties
It’s important to note that strings have an important property known as immutability. This means that once a string is created, the elements within it can not be changed or replaced. For example:
s
'Hello World'
Let’s try to change the first letter to ‘x’
s[0] = 'x'
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
<ipython-input-26-976942677f11> in <module>()
1 # Let's try to change the first letter to 'x'
----> 2 s[0] = 'x'
TypeError: 'str' object does not support item assignment
Notice how the error tells us directly what we can’t do, change the item assignment!
Something we can do is concatenate strings!
s= s + ' concatenate me!'
print(s)
'Hello World concatenate me!'
we can use the multiplication operator to achieve repetition of elements.
letter = 'z'
letter*10
'zzzzzzzzzz'
Basic Built-in String methods
Python objects often have pre-defined methods that come as part of the object. These methods, which are essentially functions within the object, can perform various operations on the object itself. To access these methods, we use the dot notation, followed by the method name. The general syntax for using methods is as follows:
object.method(arguments)
Where the arguments are optional parameters that can be passed to the method. If some parts of this explanation are not clear at this moment, don’t worry, we will delve into it further when we start creating our own objects and functions.
Below are a few examples of built-in methods for strings:
s
'Hello World concatenate me!'
To change to uppercase:
s.upper()
'HELLO WORLD CONCATENATE ME!'
To change to lowercase:
s.lower()
'hello world concatenate me!'
Split a string by blank space (this is the default):
s.split()
['Hello', 'World', 'concatenate', 'me!']
Split by a specific element (doesn’t include the element that was split on)
s.split('W')
['Hello ', 'orld concatenate me!']
There are many more methods than the ones covered here. we will cover it on future posts
Print Formatting
We can use the .format() method to add formatted objects to printed string statements.
The easiest way to show this is through an example:
'Insert another string with curly brackets: {}'.format('The inserted string')
'Insert another string with curly brackets: The inserted string'
We will discuss other options for print formatting in future articles.