Numbers and airthmetic Operators in Python
Basic types of Numbers and Arithmetic Operations in python
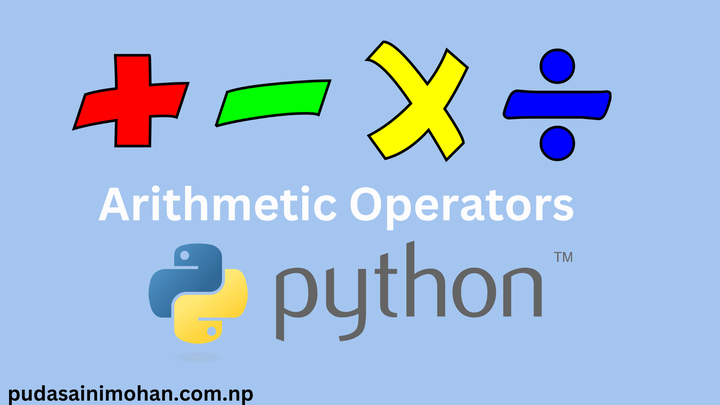
Python offers a range of numeric data types, including integers, floating-point numbers, and complex numbers. In this article, our main focus will be on integers and floating-point numbers. Integers are whole numbers that can be either positive or negative, for instance 7 or -10. Floating-point numbers, on the other hand, have decimal components or use exponential (e) notation, such as 2.0, -2.1, or 4e2.
Here is a table of the two main types we will spend most of our time working with some examples:
Examples | Number "Type" |
---|---|
1,2,-5,1000 | Integers |
1.2,-0.5,2e2,3E2 | Floating-point numbers |
Now let’s start with some basic arithmetic.
Basic Arithmetic
Python provides several built-in arithmetic operators for basic calculations, including: addition (+), subtraction (-), multiplication (*), division (/), and modulo division (%). Additionally, it provides the exponentiation operator (**) which computes the power of a number and the floor division operator (//) which returns the integer quotient of division. Here are some examples of each of these operators:
Addition
7+4
11
Subtraction
7-4
3
Multiplication
7*4
28
Division
7/4
1.75
Floor Division:
7//4
Unexpected result from 7 divided by 4 equals 1, not 1.75. This is because of the use of floor division (// operator). It returns the integer result by truncating the decimal.
What if we just need the remainder?
7%4
3
4 goes into 7 once, with a remainder of 3. The % operator returns the remainder after division. let’s continue with some other operators.
Exponential
2**3
The expression 2**3 in Python calculates 2 raised to the power of 3, which equals 8. The ** operator is used for exponentiation in Python. similary we can use power 0.05 to calculate the square root.
4**0.5
2.0
Variable Assignments
With a basic understanding of numbers in Python, let’s move on to assigning labels to values through the use of variables. To create a variable, you simply use a single equals sign (=) followed by the value you want to assign. Let’s explore a few examples to illustrate this process.
a = 10
We have created an object with a value of 10. If we reference the object, designated as a, within our Python script, Python will recognize it as the number 10
a+a
20
What happens on reassignment? Will Python let us write it over?
a = 15
let’s check
a
15
Python lets you reassign variables with a reference to the same object.
a=a+15
a
30
There’s actually a shortcut for this. Python lets you add, subtract, multiply and divide numbers with reassignment using +=
, -=
, *=
, and /=
.
a += 15
a
45
Yes! Python allows you to write over assigned variable names. We can also use the variables themselves when doing the reassignment.
When creating variables or objects in Python, certain guidelines must be followed to ensure their names are valid. This includes:
- Starting the name with a letter, not a number.
- Utilizing underscores(_) instead of spaces.
- Avoiding special characters like ‘’, “, <, >, /, ?, |, , (, ), !, @, #, $, %, ^, &, *, ~, -, and +.
- Keeping names in lowercase, as recommended by PEP8.
- Steering clear of single letter names such as l, O, and I which can be easily misconstrued.
- Refraining from using words that hold special meaning in Python, for instance, “list” or “str”.
Assigning values to variables in Python can greatly aid in organizing and tracking the data in your code. Here’s an example
my_salary = 50000
tax_rate = 0.13
total_tax = my_income*tax_rate
By accessing the total_tax variable, we can easily calculate the tax amount:
total_tax
6500.0